Python Functions And Modules: A Beginner’s Guide
Python Functions And Modules: A Beginner’s Guide
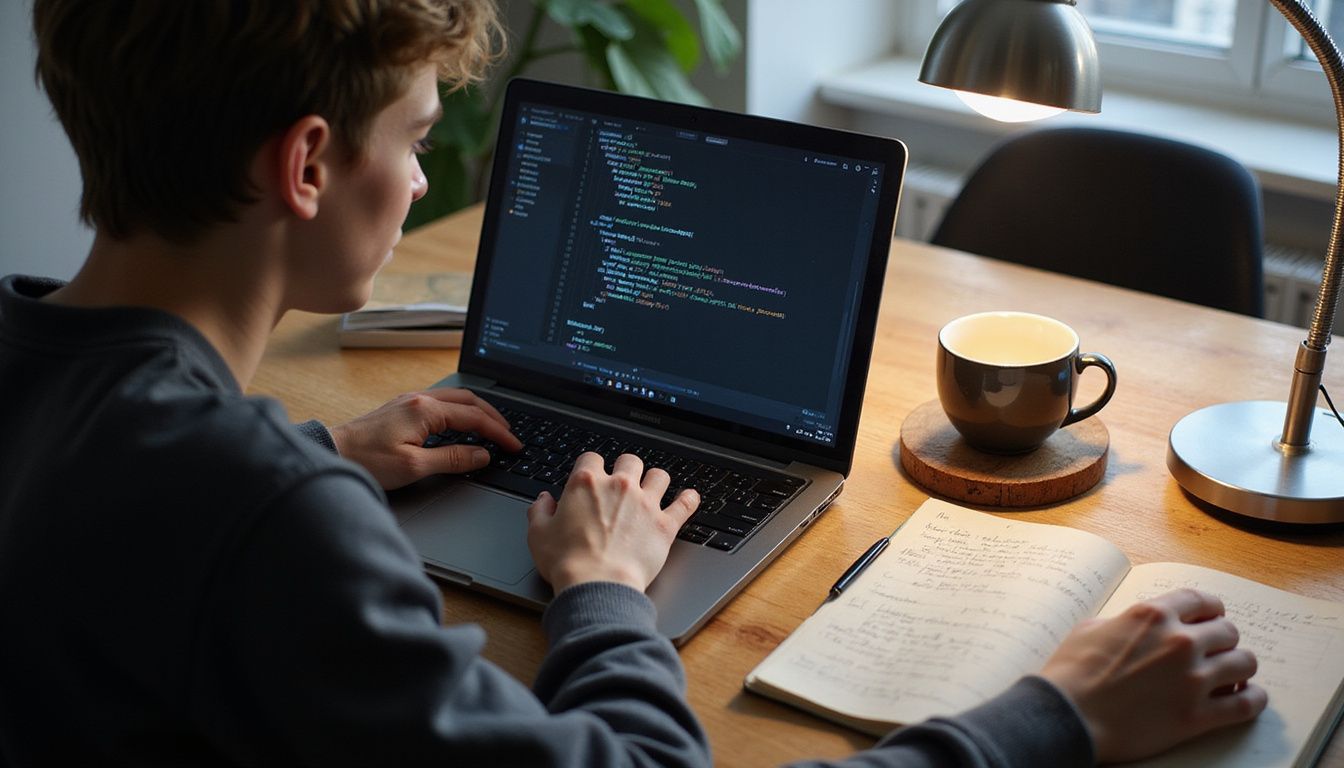
Are you trying to make your own website or software but find coding confusing? You might have heard about Python. It’s a tool that can help simplify things for you. Python uses something called “Python Functions” to make tasks easier.
Python is free and great for beginners. At Loopfinite, we’ll show you how using functions and modules in Python can be a big help in coding. Our guide is easy to follow and will teach you the basics step by step.
Keep reading to learn more!
Key Takeaways
- Python functions are blocks of code that do specific tasks and make programming easier. You can use built-in, user-defined, anonymous, and recursive types.
- You can create a function with the “def” keyword and call it using its name followed by parentheses. Functions can return values with the “return” statement.
- Variables in functions have local scope, meaning they only work inside their function. Global variables work anywhere in the program.
- Recursive functions let a function call itself to solve problems. Lambda expressions or anonymous functions are small one-time use functions for simple tasks.
- Nested functions allow you to define a function within another function for better code organization and readability.
Python Functions: An Overview
A Python function is a block of code that performs a specific task when called. Functions play a crucial role in organizing and streamlining Python programming tasks.
What is a Python Function?
A Python function is a block of code that does a specific task. You write it once and use it many times. These functions help make our code shorter and more readable. Python has different kinds of functions like built-in, user-defined, anonymous, and recursive functions.
User-defined functions let people create their own tasks in code.
Using these functions makes programming easier and helps in building websites or automating tasks. They are key parts of learning Python for beginners because they teach how to organize and reuse code efficiently.
By understanding how to use them, you can start making your own programs quickly.
Importance of Functions in Python Programming
Functions in Python make coding easier and faster. They help to break down complex problems into simpler tasks. This makes programs shorter, easier to read, and easier to update. Functions also avoid repetition by allowing the same code block to be used many times throughout a program.
This is crucial for beginners learning programming with Python’s efficient high-level data structures.
Python supports different types of functions such as built-in, user-defined, anonymous, and recursive functions. Each type serves unique purposes in coding from performing common tasks to creating custom behavior or carrying out repeated operations without writing the same code multiple times.
Using functions effectively can significantly enhance productivity and efficiency in web development or data analysis projects.
Creating and Defining Functions
Creating and defining functions is an essential part of Python programming. You can define a function using the syntax and then use it whenever needed in your code.
Syntax for Defining a Function
To define a function in Python, start with the keyword “def” followed by the name of the function. Then add parentheses () and a colon :. Inside parentheses, list any parameters your function will take.
Make sure to indent the block of code that forms the body of the function. This is where you write what your function should do.
For example, if you want to make a simple function named greet, type `def greet():`. On the next line, indented, add `print(“Hello!”)`. This tells Python that whenever you call “greet()”, it should display “Hello!”.
Example: Creating a Simple Function
Creating a simple function in Python involves defining the function using the “def” keyword, followed by the function name and parentheses. Within the parentheses, you can specify any parameters the function will take, if necessary.
The body of the function is then indented below with one or more statements that define what the function does. For example:.
python
def greet():
print(“Hello! Welcome to Loopfinite.”)
In this example, we’ve created a basic function called “greet()” which simply prints out a greeting message. This allows you to reuse this code anywhere in your program by simply calling “greet()”.
Calling Functions in Python
When you need to use a function you’ve defined, simply use its name followed by parentheses. For example, if you have a function called “calculate_total,” you’d write “calculate_total().” You can also pass values into the function by placing them within the parentheses when calling it.
How to Call a Function
To call a function in Python, you simply use the function’s name followed by parentheses. If the function has any parameters, you provide them within the parentheses. For example, if you have a function called “add_numbers” that takes two arguments, you would call it like this: add_numbers(5, 3).
This would execute the “add_numbers” function with the arguments 5 and 3.
You can also define functions to return values using the “return” statement so that when you call the function, it will give back a result or perform a specific action. Using PyCharm for Python development can greatly assist in calling functions efficiently and help beginners learn how to properly utilize Python’s capabilities.
Understanding how to call functions is an important step in mastering Python programming fundamentals and creating effective web development solutions.
Next: Function Arguments and Parameters
Example: Function Call with Parameters
When calling a function with parameters in Python, you provide the necessary arguments within the parentheses. For instance, consider a function named “multiply” that takes two parameters: num1 and num2.
To call this function with specific values for these parameters, you’d write “multiply(5, 3)” where 5 is passed as num1 and 3 as num2. This enables the function to perform its task using these specified values.
The ability to pass arguments when calling functions provides flexibility and customization, allowing functions to work with different sets of data based on what’s provided during the call.
Understanding how to effectively use parameters when calling functions is an essential aspect of Python programming for beginners seeking to enhance their coding skills and create more robust applications.
Function Arguments and Parameters
Python functions use different types of function arguments such as positional, keyword, default, arbitrary (*args), and arbitrary keyword (**kwargs). These help provide flexibility in passing parameters to functions based on specific needs.
Types of Function Arguments
Function arguments in Python can be classified into several types, each serving a specific purpose. The first type is positional arguments, where the order of input matters when passing values to a function.
Then there are keyword arguments, allowing us to explicitly name parameters when calling a function. Default arguments come next, providing pre-set values for parameters if no argument is passed by the user.
After that are arbitrary arguments (*args), which enable functions to accept an arbitrary number of positional arguments. Lastly, we have arbitrary keyword arguments (**kwargs), which allow functions to accept an arbitrary number of keyword arguments.
Understanding these different types of function arguments is crucial for effectively utilizing Python’s flexibility and enhancing coding efficiency in web development and beginner programming scenarios.
Positional Arguments
Positional arguments play a fundamental role in Python functions. They enable the passing of arguments based on their position, signifying the significance of the order in which the arguments are passed.
For instance, if a function anticipates two positional arguments, they need to be supplied in the precise order as stipulated in the function definition. This is valuable when supplying input values to functions and contributes to the development of adaptable and reusable code.
Grasping the functionality of positional arguments is imperative for mastering Python functions and modules, empowering beginners to craft more intricate and productive programs.
Transitioning from positional arguments, let’s explore “Keyword Arguments” – another pivotal facet of Python functions that confers flexibility in parameter passing.
Keyword Arguments
In Python, keyword arguments enable you to pass values to a function using parameter names. This method allows you to assign values to specific parameters when calling the function, without being dependent on the parameter order in the function definition.
For instance, a function with parameters like “name,” “age,” and “city” can be called using keyword arguments such as “city=’New York’,” “name=’John’,” and “age=25.” This simplifies understanding and correspondence between arguments and parameters.
Mastering the effective use of keyword arguments is crucial for beginners as it improves code readability and minimizes errors. Grasping the functionality of keyword arguments provides beginners with greater flexibility and control over their functions’ behavior as they progress in learning Python functions and modules.
Next, let’s explore Default Arguments.
Default Arguments
Now let’s talk about default arguments. In Python, a default argument is a parameter that assumes a default value if a value is not provided by the function call for that argument.
This is particularly useful when you want to define a function with some pre-set values but also allow the flexibility for users to input their own values.
For instance, if you have a function that multiplies two numbers and the second number has a default value of 2, users can choose whether they want to provide their own second number or just use the default one.
This makes functions more versatile and user-friendly.
Arbitrary Arguments (*args)
Arbitrary Arguments (*args) offer the flexibility of handling a variable number of function arguments, making it convenient when the exact count of inputs is unknown. When developing a function to calculate the sum of numbers, utilizing *args allows for processing multiple inputs without explicitly specifying each one.
It’s akin to having a flexible container for input values.
The use of Arbitrary Arguments (*args) in Python functions highlights the language’s adaptability and versatility for diverse programming requirements. By integrating *args into your functions, you can improve efficiency and adapt your code to various scenarios, aligning with Python’s reputation as a beginner-friendly yet potent language for web development and beyond.
Arbitrary Keyword Arguments (**kwargs)
In Python, **kwargs allows you to pass a variable number of keyword arguments to a function. This can be useful when you’re uncertain about the number of keyword arguments that will be passed to your function.
The double asterisks in front of kwargs allow you to take in such variables and store them as dictionary elements within the function.
Using **kwargs provides flexibility by enabling functions to accept any number of keyword arguments, making your code more adaptable and easier to maintain especially for web development tasks.
Loopfinite makes it easy for beginners with its simple and clear explanations, guiding users through understanding and implementing these concepts effectively in their Python programming journey.
Return Statement in Functions
The return statement in functions allows you to send a value back after the function completes its task.
It’s a crucial part of Python functions as it helps pass on important data from the function for further use.
Using the return Statement
The return statement in Python functions is used to hand back a value after the function completes its task. It allows the function to send data back to the caller. For instance, if a function calculates a sum, it can use the return statement to provide that result so it can be used elsewhere in the code.
Understanding how and when to use this feature is crucial for writing effective and reusable functions.
It’s crucial for beginners learning Python functions and modules through Loopfinite tutorials to grasp how returning values from functions with the ‘return’ statement enhances their ability to create efficient and modular code.
This not only helps them comprehend programming fundamentals but also paves the way for a deeper understanding of building packages and organizing Python code effectively.
Example: Function with Return Value
To understand how a function returns a value in Python, let’s consider an example. Suppose we have a function called “add_numbers” that takes two arguments, x and y. In this function, we can use the “return” statement to give back the result of adding x and y together.
For instance:.
python
def add_numbers(x, y):
sum = x + y
return sum
Here, when we call the “add_numbers” function with values 3 and 4 like this: “result = add_numbers(3, 4)”, it will return the result which is 7. This returned value can be further used or stored for later use in our code.
Understanding how to use the return statement is crucial as it allows functions to provide results for other parts of your program or perform actions based on those results. It’s an essential aspect while learning about Python functions and modules.
Scope and Lifetime of Variables
Variables within a function can only be accessed inside that function. This means they have a local scope and are destroyed when the function finishes executing.
Local and Global Variables
Local variables are those that are declared within a function and can only be accessed within that specific function. On the other hand, global variables are defined outside any function and can be accessed throughout the program.
It is essential to understand the scope of these variables as it impacts their accessibility in different parts of your code. Python’s scoping rules ensure that local variables do not conflict with global ones, providing clarity and organization to your code structure.
Understanding this concept allows for efficient use of both local and global variables in Python programming, contributing to clean and manageable code development.
Scope of Variable within Functions
Local and global variables in Python have different scopes. A local variable is accessible only within the function where it’s defined, while a global variable can be accessed throughout the program.
When using local variables within a function, they are created when the function is called and destroyed when the function exits. On the other hand, global variables are defined outside of functions and can be used anywhere in the program.
Understanding this scope of variables is essential for writing efficient and organized Python code.
Recursive Functions
Recursive functions involve a function calling itself, creating a loop of repeated actions. For example, you can use recursive functions to solve problems that can be broken down into smaller repetitive tasks.
What is Recursion?
A recursive function is a type of function in Python that calls itself during its execution. Unlike iterative functions, recursive functions use the concept of self-reference to solve problems.
In simpler terms, recursion involves breaking down a problem into smaller sub-problems and solving each sub-problem using the same method. This process continues until a base condition is met, which stops the function from calling itself further.
Recursion is particularly useful for tasks such as searching, sorting, and traversing data structures like trees and graphs because it can simplify complex problems into more manageable parts.
For instance, when you need to calculate factorials or Fibonacci numbers in Python programming, using recursion often results in concise and elegant code compared to iterative solutions.
However, it’s important to note that while recursion offers an efficient way to solve certain problems in Python programming, too much recursion can lead to performance issues due to excessive memory consumption.
Therefore, understanding how and when to use recursion effectively is crucial for beginner programmers learning Python functions and modules.
Example: Recursive Function
Now, let’s see an example of a recursive function in Python. A recursive function is a function that calls itself within its own definition. It’s commonly used to solve problems involving smaller instances of the same problem.
For instance, one popular example of recursion is the factorial function which calculates the product of all positive integers from 1 to n where n is a given number. It can be implemented through a simple recursive approach.
The following code depicts how to create a recursive function for calculating the factorial:
python
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n – 1)
In this code, once ‘factorial’ is called with an argument ‘n’, it multiplies ‘n’ with the result obtained from calling ‘factorial’ with argument ‘(n-1)’. This process repeats until it reaches base case (i.e., when ‘n’ becomes zero).
This illustrates how recursion works in Python programming and demonstrates its practical usage.
Anonymous Functions: Lambda Expressions
Lambda expressions are concise and powerful. They offer a way to create small, anonymous functions. These can be useful when you need a simple function for a short period of time.
Introduction to Lambda Expressions
Lambda expressions are a concise and powerful way to create small anonymous functions in Python. These functions can have any number of arguments but can only contain one expression.
Lambda functions are useful when used as an argument for higher-order functions like map(), filter(), and reduce(). They provide an elegant way to perform operations using fewer lines of code.
For instance, using lambda expressions with the map() function can efficiently apply a specific operation to all elements in a list, making it valuable for beginners learning Python programming.
Lambda expressions are particularly handy when working with Python’s functional programming tools and can enhance the readability of your code by simplifying it. Mastering lambda expressions is key for those getting started with Python development, especially when exploring Loopfinite’s beginner-friendly guide on Python Functions & Modules.
Example: Using Lambda Functions
Transitioning from the introduction to lambda expressions, we now illustrate how to use lambda functions in Python. Lambda functions are concise and single-use functions often used for small tasks.
For instance, a simple lambda function can be written as “lambda x: x ** 2” which squares a given number ‘x’. These anonymous functions are commonly passed as arguments to higher-order functions like map(), filter(), or reduce().
They provide a quick and efficient way to perform operations without needing to define a formal function.
Lambda functions offer simplicity and brevity in code, making them useful for processing data on-the-fly within lists or other iterables. Their flexibility allows for coding with fewer lines, enhancing readability and maintaining the focus on specific task-based operations within your Python programs.
Nested Functions
Nested functions involve defining functions within other functions. It allows for creating modular and organized code structures, leading to better code readability and maintenance.
Defining Functions within Functions
A function inside another function is called a nested function. This kind of setup helps in organizing code to improve readability and reuse code blocks efficiently. Loopfinite encourages using this method for more organized and maintainable Python programming.
It allows developers to keep their main functions clean by delegating smaller tasks to separate, nested functions, which can be useful when handling complex logic or repetitive tasks.
Example: Nested Function
A nested function in Python is a function defined within another function. This allows the inner function to access the variables of the outer or enclosing function, which can be useful for reusability and maintaining clean code.
An example of this would be a situation where an outer function performs a specific task that requires additional sub-tasks to be carried out by an inner function, ensuring better organization and modularity within the program.
Understanding and implementing nested functions can greatly enhance one’s grasp of Python programming while streamlining their coding practices, especially when working on more complex projects related to web development or software creation.
In addition to helping organize code, nested functions provide flexibility in managing data and operations within different layers of a program. They are valuable tools for creating efficient algorithms and building robust applications in Python without adding unnecessary complexity.
Developing proficiency in utilizing nested functions is crucial for aspiring programmers seeking to strengthen their foundation throughout their learning journey with Python functions and modules.
Python Modules: An Overview
Python modules serve as an essential part of organizing code and enhancing reusability. They allow for the creation and management of efficient, modular, and reusable code segments that can be utilized across multiple projects.
What is a Python Module?
A Python module is a file containing Python definitions and statements. It’s a way to organize related code into a single file. Modules can define functions, classes, and variables and are reusable when you have several scripts that share similar functionality.
Python modules play an essential role in structuring programs. They help manage complexity by breaking large programs into smaller, more manageable parts. These modules enhance reusability and maintainability of the code, making it easier to collaborate with other developers in projects.
Importance of Modules in Python
Modules are an essential part of Python programming, offering a way to organize and structure code effectively. They help in reducing complexity and enhancing reusability by breaking down large programs into smaller, manageable parts.
With modules, different functionalities can be separated into distinct files, allowing for easier maintenance and troubleshooting. For example, when building web applications with Python for Loopfinite’s web development needs, modules play a crucial role in organizing code related to server-side scripting or other specific functions.
Moreover, modules allow you to tap into the extensive collection of pre-written code available in the Python Standard Library and third-party libraries. This enables developers at Loopfinite to leverage ready-to-use solutions for tasks like data manipulation, mathematical operations, networking protocols, or even graphical user interfaces.
By using standard library modules tailored towards web development needs as well as creating custom user-defined modules unique to Loopfinite’s projects demands, it helps streamline the coding process while maintaining high quality throughout its suite of services.
Creating and Importing Modules
In Python, you can create your own modules by defining functions and variables in a separate file. You can then import these modules into your main program using the “import” keyword.
How to Create a Module
To develop a module in Python, individuals can write functions and variables in their code, save it with a .py extension, and then import it into other Python files. This enables the organization of code into reusable units, promoting effectiveness and clarity.
For example, when crafting a personalized module named “example_module.py” with specific functions such as add() and subtract(), one would subsequently be able to import this module using Loopfinite’s provided approaches.
By doing so, one can efficiently distribute and reuse code across various projects without redundant efforts or compromising quality.
Moreover, through the practice of creating tailored modules and methodically importing them within their work environment as guided by Loopfinite, newcomers are encouraged to adopt strong techniques that not only improve productivity but also showcase competency in coding literacy vital in modern web development scenarios.
Importing a Module in Python
To include a module in Python, you can utilize the “import” keyword followed by the name of the module. For instance, to include the math module, you can write: “import math”. This permits you to access all the functions and variables defined in the math module within your code.
Moreover, you can also use an alias when including a module. This is helpful when working with modules with long names or when there’s a chance of name conflicts. For example, if you want to import the math module but refer to it as “m” in your code, you can write: “import math as m”.
This way, whenever you need to use a function from the math module, you would simply preface it with “m.” instead of “math.”.
Built-in Python Modules
13. Built-in Python Modules are pre-made tools that provide additional functionality to your Python programs, allowing you to perform complex tasks without having to write the code from scratch.
These modules include commonly used functionalities like math and datetime, making it easier for developers to access essential features in their projects.
Overview of Common Built-in Modules
Python comes with a range of built-in modules that provide ready-to-use functions and tools to simplify programming tasks. One such module is the ‘math’ module, which offers mathematical functions like trigonometric operations, exponentiation, logarithmic functions, and numerical constants.
Another significant one is the ‘random’ module, enabling the generation of random numbers for various applications such as simulations or games. The ‘datetime’ module allows easy manipulation of dates and times in Python programs, while the ‘os’ module provides functions for interacting with the operating system.
These built-in modules greatly enhance Python’s capabilities by offering essential functionalities right out of the box without demanding additional installations or configurations.
They are fundamental resources for beginner programmers seeking to develop efficient code and explore diverse applications within Python development.
Example: Using the math Module
Now, let’s apply what we’ve learned. Python’s built-in math module is a valuable tool for performing mathematical operations in our programs. For instance, to determine the square root of a number, we can easily import the math module and utilize its sqrt() function.
This simplifies intricate mathematical calculations significantly. The math module also offers functions for trigonometric, logarithmic, and other common mathematical operations, which can be incredibly beneficial in various web development projects.
Understanding how to use the math module effectively, beginners learning about Python functions and modules will gain practical insights into implementing advanced functionalities within their code.
It demonstrates how leveraging existing modules can streamline coding processes and enhance overall program efficiency without needing to reinvent the wheel.
User-Defined Modules
Learn how to create custom modules with examples in Python. Importing a Custom Module: Understand the process of importing and using a custom module in your Python code.
Creating Custom Modules
Developing custom modules in Python is vital for organizing and reusing code efficiently. By developing your own modules, you can encapsulate functions and variables specific to your project’s requirements.
For example, if you’re creating a website for Loopfinite, you could develop a personalized module to manage user authentication and database interactions. This enhances the modularity of the code and simplifies maintenance.
Moreover, through the use of user-defined modules such as those at Loopfinite, developers can conceal the implementation details of their code within these modules, enabling better abstraction and separation of concerns.
It also fosters collaboration as these personalized modules can be shared across various projects or with other developers working on similar tasks. Understanding how to develop custom Python modules is crucial for constructing scalable and maintainable web applications.
Now, let’s explore the process of importing a Custom Module.
Example: Importing a Custom Module
Creating custom modules is a useful aspect of Python programming, particularly when developing web applications. By creating custom modules, developers at Loopfinite can organize their code into reusable components, making it easier to manage and maintain.
For instance, if there are specific functions or classes that are repeatedly used across different parts of the application, they can be grouped into a module for easy access. Moreover, importing a custom module in Python is straightforward; it involves using the import statement followed by the name of the module.
To elaborate on this further – Let’s move on to understanding “Overview of Common Built-in Modules”.
Python Standard Library
Python Standard Library includes a comprehensive collection of modules that provide ready-to-use functionalities for various programming tasks. Explore the wide range of pre-built modules to streamline and enhance your Python programming experience.
Overview of the Python Standard Library
The Python Standard Library is a collection of modules and packages that come with Python installation. It provides a wide range of functionalities, including file I/O, system calls, sockets, and even internet protocols.
Many standard library modules are particularly useful for web development tasks.
For example, the ‘http.server’ module can be used to set up simple web servers and test web applications locally. The ‘json’ module allows easy encoding and decoding of JSON data. Moreover, the ‘urllib’ module helps in opening URLs using a variety of protocols.
These built-in tools save time for developers as they don’t have to write these functionalities from scratch. Loopfinite understands the importance of leveraging this rich collection when developing web applications or software solutions in Python language.
Useful Standard Library Modules for Web Development
The Python Standard Library offers several useful modules for web development. One such module is the “http” module, which provides tools for constructing HTTP servers and clients.
It enables you to manage requests, handle cookies, and implement authentication within your web applications.
Another important module is “urllib”, which allows you to access URLs and interact with webpages by fetching data from them or submitting data to them. This can be helpful when your web development project involves accessing external resources or APIs.
In addition, the “json” module is advantageous for managing JSON data commonly used in modern web development. It offers functions for encoding and decoding JSON data, simplifying the process of working with APIs that communicate using this format.
Furthermore, the “cgi” module helps create dynamic web content by processing information submitted via HTML forms on the server side. It’s particularly valuable when managing user input on websites designed using Loopfinite’s programming expertise.
Best Practices for Using Functions and Modules
When using Python functions and modules, write clear and well-documented code to make it easier for others to understand. Document your functions and modules thoroughly to ensure they can be reused efficiently.
Writing Clean and Reusable Code
Writing efficient Python code relies on creating clean and reusable code. Well-structured and well-documented code facilitates maintenance, debugging, and code reusability. Adhering to best practices, like using descriptive variable names and comments, makes it easier for beginners to comprehend code written by others or themselves later on.
Furthermore, maintaining consistent formatting standards ensures readability across different modules or functions within a project.
To achieve clean and reusable Python code, beginners should strive for modularization – breaking down the program into smaller, specific parts. This allows each module to be independently reused in different parts of the program without duplicating similar tasks.
Additionally, clearly documenting functions and modules will aid other developers in understanding their purpose and effective utilization.
Developers should also focus on adhering to PEP 8 guidelines – the style guide for Python coding promoting consistency across projects. Grasping these principles early simplifies collaboration among developers when working on shared projects, while cultivating good coding habits from beginner levels onward.
Documenting Functions and Modules
When working with Python functions and modules, it is essential to document them effectively. Clear documentation helps in understanding the purpose, input parameters, and expected output of a function or module.
This becomes particularly important when collaborating on projects or when revisiting code after some time.
Thorough documentation also aids in maintaining clarity for future reference. By providing detailed explanations within the code itself, developers can ensure that others (or even their future selves) have an easier time understanding and utilizing the functions and modules created.
Additionally, well-documented functions and modules contribute to building a reliable library of reusable code, enabling efficient development processes for web solutions. It is crucial to prioritize clear and concise documentation as an integral part of the programming journey.
Conclusion
– Python Functions & Modules: A Beginner’s Guide equips you with fundamental skills in Python programming.
– You’ve learned how to create and utilize functions, grasp the significance of modules, and understand variable scope.
– With Loopfinite as your guide, you’re poised for success in programming using Python.