Python Syntax For Beginners: Variables, Data Types And Operators
Python Syntax For Beginners: Variables, Data Types And Operators
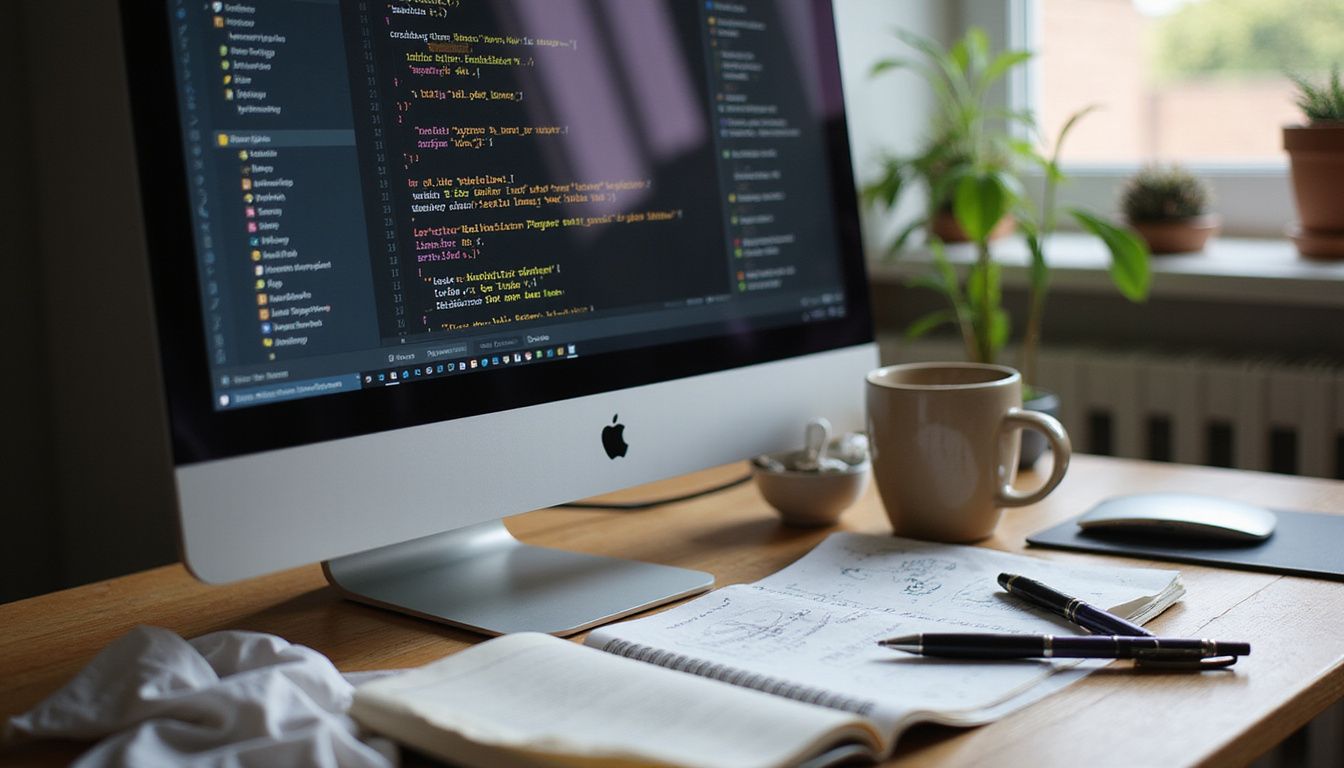
Starting to program in Python can feel like a big step. You might not know where to begin. This is common, especially when you’re trying to understand Python syntax for beginners. One interesting thing about Python is that its data types are classes, and variables are instances of these classes.
This fact makes Python powerful.
At Loopfinite, we want to make learning easy for you. Our guide covers everything from variables, data types, to operators in simple terms. We break down the basics so you can start coding quickly.
Ready to learn more? Keep reading!
Key Takeaways
- Python uses simple rules for variables and naming. Variables are like containers for data. Names must start with a letter or underscore.
- There are many types of data in Python, such as numbers, text (strings), and true/false values (Booleans). Also, there are lists, tuples, sets, and dictionaries for organizing data.
- Operators in Python help change data. These include doing math (+,-,* etc.), comparing things (>,<,== etc.), and combining conditions (and, or).
- Indentation makes the code clean and easy to read. It shows where parts of the code start and end.
- Comments in the code make it easier to understand. They can explain what part of the code does without affecting how it runs.
Understanding Python Syntax
Moving from an introduction to the core of Python programming, understanding its syntax is like learning the alphabet before you can read. The basics of Python syntax involve writing code that computers understand.
You use variables to handle data in memory. This process forms the foundation for all programming tasks in Python.
Python syntax has rules for how you write your code. For example, each variable is an object and part of a class. This means when you create a variable, you are making an instance of a class.
Learning these rules helps beginners manipulate different types of data like numbers, strings, and lists effectively. With practice, anyone can get good at using Python’s operators for math operations and more complex tasks.
Python Variables
Python Variables are used to store data, follow naming rules, and are assigned values. They are the building blocks of Python programming.
Defining Variables
Forming variables in Python implies instructing the computer to store a particular value with a specific name. Consider variables as containers where you can place items like numbers, words, or lists.
To establish a variable, you simply assign it a name and then designate what it should hold. As an illustration, to maintain a record of your age, you might code `age = 30`. In this scenario, `age` is the variable name, and `30` is the value it contains.
As each variable in Python is an entity, this phase facilitates work with a plethora of data types. Variables serve as markers that reference these pieces of data stashed in the computer’s memory.
They simplify programming by enabling us to employ names rather than memorizing sophisticated addresses for our data. Regardless of whether we’re working with numbers or words (strings), setting up variables correctly is crucial for efficient data manipulation and access in Python programs.
Variable Naming Rules
After learning how to define variables in Python, the next step is understanding the rules for naming them. Names must start with a letter or an underscore. They can’t begin with numbers.
You can include letters, numbers, and underscores in the rest of the name. Python is case sensitive, which means “Variable” and “variable” would be seen as two different names.
Keep names descriptive yet short to help others understand your code better. Avoid using Python’s built-in function names as variable names; this could cause unexpected errors in your programs.
Stick to these simple guidelines to make sure your code runs smoothly and is easy for others to read.
Assigning Values
Python enables the assignment of values to variables using the equal sign. For instance, “x = 5” assigns the value 5 to the variable x. This is a fundamental concept in Python, where variables serve as containers for holding data.
In Python, the value assigned to a variable can be changed at any point in the code. For example, if you initially set x to 5 and later assign it a new value of 10 with “x = 10”, Python will accordingly update the value stored in x.
This adaptability makes programming more dynamic and adaptable when dealing with data and computations.
This characteristic equips programmers with versatile tools for efficiently managing and manipulating data within their programs. It also empowers them to create applications that can dynamically respond to changing input or conditions, ultimately enhancing the overall functionality of their software solutions.
Data Types in Python
Python supports various data types such as integers, floats, strings, booleans, lists, tuples, sets and dictionaries. Explore the depths of Python’s data versatility.
Numeric Types: Integers and Floats
Python’s numeric types include integers and floats. Integers are whole numbers, while floats are numbers with decimal points. Variables in Python can hold both types of numeric values, and the language supports basic mathematical operations such as addition, subtraction, multiplication, and division for these numeric types.
It is important to note that Python provides a wide range of data manipulation capabilities for these numeric types through built-in functions and operators. Understanding the distinctions between integers and floats is fundamental when learning Python programming.
Strings
Moving on from understanding numeric types like integers and floats, let’s explore another essential data type in Python – Strings. In Python, strings are used to represent text rather than numbers.
They consist of sequences of characters, enclosed within single or double quotation marks. Strings can be modified using a variety of operations and functions, making them a fundamental aspect of programming.
Python facilitates straightforward modification of strings through joining (concatenation), extracting parts (slicing), and inserting variables into strings (formatting). Mastering the manipulation of strings is vital for effectively handling textual data in Python programming language.
Booleans
Moving from understanding strings to Booleans, it’s essential for beginners to grasp the concept that Booleans are a data type in Python representing truth values. They are denoted as either True or False and play a crucial role in decision-making within programs.
Python relies on Booleans to execute conditional statements, control flow, and logical operations.
Python provides built-in support for Booleans, allowing programmers to create expressions that evaluate to True or False based on specific conditions. This feature significantly enhances the language’s capability in handling decision-making processes within scripts and programs effortlessly.
Understanding how to effectively utilize Boolean logic is fundamental for beginners learning Python programming.
Lists, Tuples, Sets, and Dictionaries
Now, let’s move on from the discussion about Booleans and shift our attention to understanding Lists, Tuples, Sets, and Dictionaries. In Python, these data structures play a vital role in organizing and managing data efficiently.
Lists are ordered collections that allow for easy modification and retrieval of items. Tuples are similar to lists but are immutable, meaning their values cannot be changed after creation.
On the other hand, sets consist of unique elements with no specific order and do not allow duplicate values. Lastly, dictionaries are used to store key-value pairs which enable fast retrieval based on keys.
Python learners should grasp the significance of these data structures since they form an essential part of Python programming basics. Understanding how to work with lists, tuples,csets,dictionaries is crucial for manipulating data effectively in various applications or projects.
Python Operators
Python operators handle data manipulation.
They include arithmetic, comparison, logical, and assignment operations.
Arithmetic Operators
Python offers a range of arithmetic operators for basic mathematical tasks. These consist of addition, subtraction, multiplication, division, modulus (remainder), and exponentiation.
For example, the addition of 5 and 3 yields 8, while subtracting 7 from 2 gives us 5.
Comprehending these core arithmetic operators is essential for conducting computations and manipulating data in Python programming.
Shifting our focus to the following segment “Comparison Operators,” let’s explore their application in Python programming.
Comparison Operators
Comparison operators in Python allow you to compare two values. They include equal to (==), not equal to (!=), greater than (>), less than (=) and less than or equal to (<=).
These operators return a Boolean value – True if the comparison is valid, and False if it is not.
The comparison operators are fundamental for making decisions in programming. For instance, you could use these operators to check whether one variable is greater or lesser than another, compare strings, or evaluate conditions within loops and conditional statements.
Understanding how these comparison operators work enables you to create more complex programs and make choices based on different conditions.
Logical Operators
Python provides three logical operators: and, or, and not. These operators are used to combine conditional statements in a Python program. The ‘and’ operator returns True if both the conditions are True.
Similarly, the ‘or’ operator returns True if at least one condition is True.
The ‘not’ operator returns the opposite of the original value; if it was False, it becomes True and vice versa. Understanding how these logical operators work is crucial for creating complex conditional statements in Python programming.
Understanding logical operators allows you to create sophisticated conditions for your Python programs that can process data effectively.
Next up is “Assignment Operators.
Assignment Operators
Assignment operators in Python are utilized to assign values to variables. For example, the statement “x = 5” assigns the value 5 to variable x using the “=” operator. This straightforward assignment operator enables novices to begin defining and manipulating variables immediately, a pivotal step in grasping Python’s syntax.
Additionally, Python offers a range of compound assignment operators like “+=”, “-=”, “*=”, “/=” and numerous others, enhancing the convenience for users to conduct arithmetic operations while reassigning values within a single expression.
Python presents adaptable assignment operators that are essential for handling variables and data manipulation. These operators play a crucial role in establishing the foundation for developers as they embark on their journey of understanding syntax rules and dynamically manipulating data types when creating code snippets or full-fledged applications.
Indentation in Python
Indentation in Python is vital for structuring code and determining the scope of functions or loops. It also ensures readability and consistency in your codebase.
Importance of Indentation
Indentation in Python is crucial as it denotes the structure of the code. It visually represents different levels of scope within functions, loops, and conditional statements. Proper indentation improves code readability and helps developers understand the program flow easily.
For example, when a new block of code starts after a colon, such as in a loop or conditional statement, it should be indented to indicate that it belongs to that block. Incorrect indentation can lead to syntax errors or unexpected program behavior.
Consistent and correct indentation practices are essential for writing clean and maintainable Python code. Adhering to established indentation rules makes the code more organized and understandable for other programmers who may work on the project in the future.
Indentation Rules
Indentation in Python is significant for code structure, where it represents the block of code. It underlines the beginning and end of a block of code, such as within loops or conditional statements.
The indentation rules are crucial to Python’s syntax and determine how the interpreter recognizes different sections of the code.
For instance, incorrect indentation can lead to errors or unexpected program behavior that might be challenging to identify. Therefore, understanding and adhering to Python’s indentation rules when writing code is fundamental for successful execution.
Adhering strictly can prevent potential issues with readability and execution order, making your programs easier to understand by yourself and others alike.
Moving on from this topic, let’s now explore “Comments in Python.
Comments in Python
Python allows for both single line and multi-line comments. Single line comments are denoted with a hash symbol (#), while multi-line comments are enclosed within triple quotes (”’).
Single Line Comments
In Python, single-line comments are created using the hash symbol (#). These comments are used to explain code or make notes for yourself and other developers. When Python encounters a #, it ignores everything following that symbol on the same line.
This makes single-line comments useful for including brief explanations within your code without affecting its functionality. For instance, in Python, you can write: # This is a single-line comment explaining the next line of code.
By including these comments in your code, you can improve readability and ensure that others can easily understand the purpose of specific lines of code.
Python emphasizes readability and simplicity; thus, using single-line comments appropriately aligns with these principles. It is recommended to use them to provide clear and concise explanations in your code wherever necessary.
Multi-line Comments
Multi-line comments in Python are essential for explaining code sequences or preventing certain lines from being executed. These comments can span multiple lines and are enclosed within three single quotes (”’).
They’re valuable for providing detailed explanations of complex code, documenting the purpose of functions or classes, or temporarily disabling a block of code during testing. For example, if you want to provide a descriptive comment regarding the functionality of a specific section of your code that spans multiple lines, multi-line comments offer an efficient way to achieve this.
As instances (objects) of these classes.
Python data types are crucial because every variable in Python is an object and it supports two types of numbers as well as arithmetic operators for basic mathematical operations like addition and subtraction.
Moreover, they are pivotal in enabling beginners to build a strong foundation in the language by emphasizing on syntax rules and type categorization alongside supporting mutable and immutable types which makes Python versatile for programming education initiatives such as those provided by Loopfinite.
Taking Input from the User
To take input from the user in Python, you can utilize the built-in function “input()”. This function allows you to prompt the user for input and store it into a variable. For example, if you want to ask for the user’s name, you can use “name = input(‘Enter your name: ‘)”.
The information entered by the user will be stored in the variable “name”, which can then be used in your program.
Using this approach, Python enables interactive communication with users by accepting their inputs directly into a program. This is particularly useful when creating programs that require dynamic or personalized data entry.
For instance, Loopfinite’s web development may involve utilizing these techniques to create interactive websites or applications that engage users through input mechanisms.
Conclusion
That’s a wrap on Python Syntax For Beginners: Variables, Data Types And Operators. Keep Loopfinite in mind to guide you through the essentials of Python programming. Whether it’s grasping variables or mastering data types and operators, we’re here to support you.
Let’s explore the world of Python together – it’s an exciting journey!